끄적끄적 코딩 공방
Hugging Face Transformers: pipeline으로 AI 모델 쉽게 사용하기
# 설치pip install transformersHugging Face의 Transformers 라이브러리는 자연어 처리(NLP), 컴퓨터 비전, 음성 인식 등 다양한 분야의 최신 AI 모델을 쉽게 활용할 수 있게 해주는 강력한 도구입니다. 그중에서도 pipeline 함수는 복잡한 모델 사용 과정을 단 몇 줄의 코드로 간소화해주는 마법 같은 기능입니다.이 글에서는 from transformers import pipeline을 활용하여 다양한 AI 작업을 손쉽게 수행하는 방법을 알아보겠습니다.pipeline의 기본 개념pipeline 함수는 모델 로딩, 전처리, 후처리 등 AI 모델 사용의 모든 단계를 추상화하여 제공합니다. 사용자는 단 몇 줄의 코드로 강력한 AI 모델의 기능을 활용할 수 있습니다.fr..
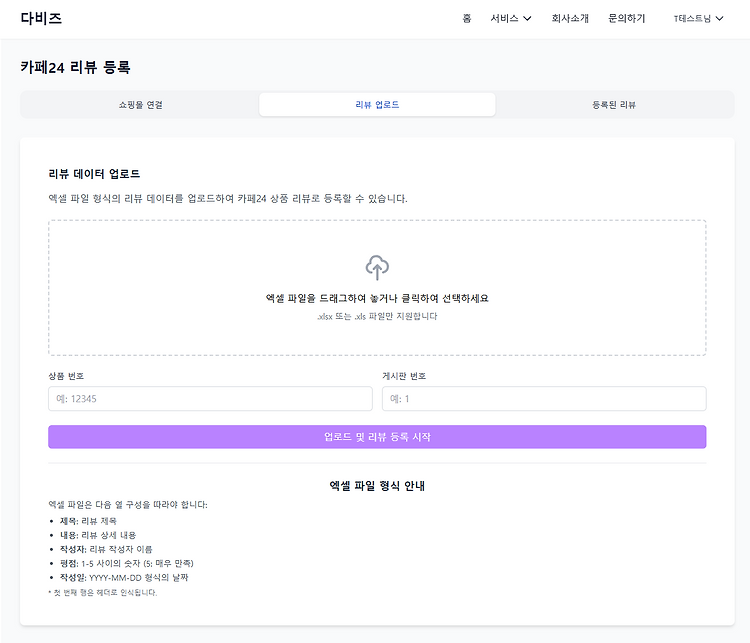
엑셀 파일 카페24 리뷰 등록
📊 리뷰가 매출에 미치는 영향, 알고 계신가요?최근 조사에 따르면, 제품 리뷰는 구매 결정에 있어 무려 93%의 영향력을 가지고 있다고 합니다. 특히 10개 이상의 리뷰가 있는 상품은 그렇지 않은 상품보다 전환율이 평균 50% 이상 높다는 사실, 알고 계셨나요?그러나 리뷰 관리는 쇼핑몰 운영자에게 항상 골치 아픈 문제였습니다.네이버 스마트스토어나 쿠팡에 등록된 리뷰를 카페24 쇼핑몰에 수동으로 옮기는 번거로움엑셀로 정리한 리뷰 데이터를 일일이 입력하는 시간 소모리뷰 작성 시 이미지 첨부와 포맷팅의 불편함 ✨ 카페24 리뷰 자동 등록 서비스 주요 기능 1. 엑셀 기반 대량 리뷰 등록네이버, 쿠팡 등 다양한 플랫폼의 리뷰를 엑셀로 추출해 한 번에 등록리뷰 내용, 별점, 작성자, 작성일 등 다양한 정보 ..
React에서 브라우저 창 제어하기: 새 창 열기부터 URL 추적까지
React 애플리케이션에서 특정 URL을 새 창으로 열고, 그 창의 변화를 추적하는 기능이 필요한 경우가 있습니다. 이 글에서는 새 창 열기, URL 가져오기, 리디렉션 감지 등 브라우저 창 제어와 관련된 다양한 방법을 알아보겠습니다.새 창 열기 기본 방법React에서 새 창을 여는 가장 기본적인 방법은 window.open() 메서드를 사용하는 것입니다.function OpenWindowButton() { const openNewWindow = () => { window.open('https://example.com', '_blank'); }; return ( 새 창에서 열기 );}window.open() 메서드는 다음과 같은 매개변수를 받습니다:첫 번째 매개변수: ..
DNS 전파와 웹 서버 접속 문제 해결 가이드
웹 서버를 설정하고 도메인을 연결했는데 접속이 안 되는 상황은 웹 개발자나 시스템 관리자가 흔히 마주치는 문제입니다. 이 글에서는 DNS 전파 시간에 대한 이해부터 포트포워딩, 방화벽 설정, 서버 구성까지 종합적인 문제 해결 방법을 살펴보겠습니다.DNS 전파(propagation)란?DNS(Domain Name System) 변경사항이 전 세계 DNS 서버에 적용되는 데 걸리는 시간을 DNS 전파 시간이라고 합니다. 도메인 설정을 변경하면 이 변경사항이 인터넷 전체에 알려지는 데 시간이 소요됩니다.DNS 전파 시간의 특징항목설명일반적인 소요 시간1-4시간최대 소요 시간최대 48시간영향 요소TTL(Time To Live) 값, DNS 제공업체, 지역별 DNS 서버DNS 전파 확인 방법# 명령줄에서 DNS ..
Next.js에서 cookies-next로 쿠키 관리하기
# 설치npm install cookies-next# 또는yarn add cookies-next# next.js 버전이 12.2~14.x 인 경우 npm install cookies-next@4.3.0 로 설치Next.js 애플리케이션에서 사용자 상태 관리나 인증 정보 저장을 위해 쿠키는 필수적입니다. cookies-next 라이브러리는 Next.js의 클라이언트와 서버 컴포넌트 모두에서 쿠키를 손쉽게 다룰 수 있게 해주는 유용한 도구입니다. 이 글에서는 cookies-next의 주요 기능과 실제 활용 방법을 살펴보겠습니다.cookies-next의 주요 기능cookies-next는 다음과 같은 핵심 기능을 제공합니다:쿠키 설정 (setCookie)쿠키 가져오기 (getCookie)쿠키 삭제 (delete..
Pydantic ConfigDict: 모델 동작 커스터마이징 가이드
Pydantic은 Python의 타입 주석을 사용하여 데이터 검증과 설정 관리를 제공하는 라이브러리입니다. ConfigDict는 Pydantic 모델의 동작을 세부적으로 제어할 수 있게 해주는 중요한 요소입니다. 이를 통해 모델 검증, 직렬화, 필드 처리 등의 다양한 측면을 커스터마이징할 수 있습니다.ConfigDict의 기본 개념ConfigDict는 Pydantic 모델의 설정 옵션을 정의하는 사전 형태의 구성입니다. 이를 통해 모델이 어떻게 작동할지 세밀하게 제어할 수 있습니다.Pydantic v1에서는 내부 Config 클래스를 사용했지만, v2에서는 model_config 클래스 변수를 통해 설정하는 방식으로 변경되었습니다.Pydantic v1 vs v2의 ConfigDict 사용법v1 방식 (내..
리눅스에서 3시간마다 인터넷 속도 자동 측정 시스템 구축하기
인터넷 속도를 정기적으로 모니터링하는 것은 네트워크 문제를 진단하고 인터넷 서비스 제공업체(ISP)의 서비스 품질을 평가하는 데 매우 유용합니다. 이 글에서는 리눅스 시스템에서 3시간마다 자동으로 인터넷 속도를 측정하고 로그를 남기는 시스템을 구축하는 방법을 상세히 알아보겠습니다.시스템 구성 요소이 시스템은 다음과 같은 구성 요소로 이루어집니다:속도 측정 도구: speedtest-cli 유틸리티자동화 스크립트: 측정 결과를 로그 파일에 기록정기 실행 설정: cron을 사용한 주기적 실행로그 관리: 로그 로테이션으로 디스크 공간 관리1. 속도 측정 도구 설치먼저 인터넷 속도를 측정할 수 있는 speedtest-cli 도구를 설치합니다. 이 도구는 Speedtest.net의 서비스를 커맨드 라인에서 이용할 ..